· 5 min read
What is the Document Object Model DOM? | Definition and Meaning
The Document Object Model DOM is a crucial concept in web development that allows developers to access and manipulate HTML and XML documents dynamically.
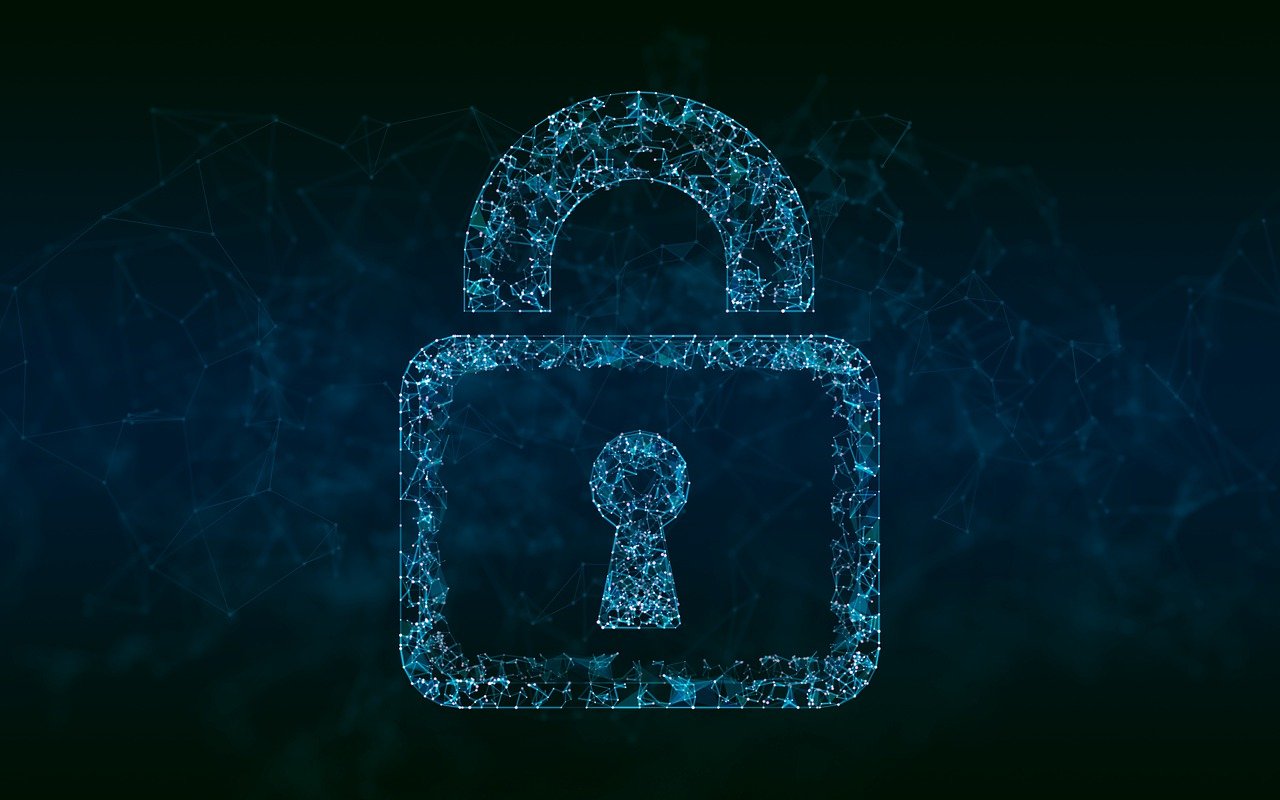
What is the Document Object Model (DOM)?
The Document Object Model, commonly abbreviated as DOM, is a crucial concept in web development that allows developers to access and manipulate the structure and content of HTML and XML documents. It represents the document as a tree of objects, enabling scripts to update the document structure, style, and content dynamically.
Understanding the DOM
At its core, the DOM is a programming interface that allows you to interact with your document in a structured way. Each element in the HTML document is represented as a node in a tree structure:
- Element Nodes: These represent HTML elements like
<div>
,<p>
, and<ul>
. - Attribute Nodes: These represent the attributes of an element, such as
class
orid
. - Text Nodes: These represent the text content within elements.
Example of DOM Structure
Consider the following simple HTML:
<div id="container">
<h1>Hello World</h1>
<p>This is a paragraph.</p>
</div>
The DOM representation of this document would look like this:
- Document
- Element (div#container)
- Element (h1)
- Text ("Hello World")
- Element (p)
- Text ("This is a paragraph.")
What does DOM mean in JavaScript?
In JavaScript, the DOM allows developers to manipulate the HTML document programmatically. For example, using JavaScript, you can modify the content of elements, change their styles, or even add new elements to the document.
Here’s a basic example of manipulating the DOM with JavaScript:
// Change the text content of the paragraph
document.querySelector('p').textContent = 'This text has been changed!';
This code selects the first <p>
element in the document and updates its text content.
The Importance of DOM Manipulation
DOM manipulation is a powerful feature that facilitates dynamic content updates without requiring a page reload. This enhances the user experience�allowing for interactive web applications and seamless updates.
Common operations involve:
- Adding elements: You can create new nodes and append them to the DOM.
- Removing elements: You can delete existing nodes.
- Changing styles: You can dynamically alter CSS styles for a more responsive layout.
- Handling events: You can add event listeners to nodes to respond to user actions (like clicks and key presses).
Practical Examples of DOM Manipulation
- Adding a new item to a list:
const list = document.querySelector('ul');
const newItem = document.createElement('li');
newItem.textContent = 'New Item';
list.appendChild(newItem);
- Removing an element:
const itemToRemove = document.querySelector('li');
itemToRemove.remove();
- Changing styles based on user interaction:
const button = document.querySelector('button');
button.addEventListener('click', function() {
const para = document.querySelector('p');
para.style.color = 'blue';
});
The Structure of the DOM Tree
The DOM tree plays a significant role in how browsers render web pages. Each node corresponds to a part of the document, and the hierarchy defines the relationships between those parts. This hierarchical structure is what enables developers to navigate and manipulate the DOM effectively.
Here’s a high-level look at the DOM tree representation:
- Root Node: The topmost node usually represents the document itself.
- Child Nodes: Nodes that fall under another node are known as child nodes. For instance, the child nodes of a
<div>
element might be its<h1>
and<p>
tags.
Diagrams and Visual Representation
Creating a document object model diagram helps in understanding how elements are nested and how they are structured. Often, you’ll see visual representations that illustrate the relationships between nodes, helping in conceptualizing how to traverse the DOM.
Known Vulnerabilities
While the Document Object Model (DOM) offers powerful capabilities for dynamic web applications, it can also introduce security risks. Various vulnerabilities related to DOM manipulation can be exploited by malicious actors if not properly managed. Below are some known vulnerabilities associated with well-known brands:
CVE-2024-0968: A cross-site scripting (XSS) vulnerability in the GitHub repository langchain-ai/chat-langchain prior to version 0.0.0. This vulnerability allows attackers to inject arbitrary scripts into web pages viewed by users.
CVE-2024-21742: This issue relates to improper input validation in the mime4j library, allowing for header injection when using the mime4j DOM for composing messages. An attacker could exploit this to add unintended headers to MIME messages.
CVE-2024-23807: The Apache Xerces C++ XML parser (versions 3.0.0 before 3.2.5) has a use-after-free error triggered during the scanning of external DTDs. Users are recommended to upgrade to version 3.2.5, or disable DTD processing to mitigate this issue.
CVE-2024-26465: A DOM-based XSS vulnerability in the component
/beep/beep.instrument.js
of stewdio beep.js before commit ef22ad7, allowing attackers to execute arbitrary JavaScript via crafted URLs.CVE-2023-0608: A XSS vulnerability found in the GitHub repository microweber/microweber prior to version 1.3.2, which can be exploited to inject malicious scripts.
CVE-2023-3010: The Grafana platform’s Worldmap Panel plugin before version 1.0.4 contains a DOM XSS vulnerability, enabling attackers to exploit the application through crafted malicious inputs.
CVE-2023-30454: An issue in ebankit before version 7 allows DOM-based XSS within the
/security/transactions/transactions.aspx
endpoint, where users can supply their JavaScript, which is then executed upon user interaction.CVE-2023-3294: A DOM XSS vulnerability was identified in the saleor/react-storefront GitHub repository prior to commit c29aab226f07ca980cc19787dcef101e11b83ef7, potentially allowing attackers to execute arbitrary scripts via user input.
These vulnerabilities underscore the importance of proper validation, encoding output, and applying security best practices when manipulating the DOM. Developers should stay vigilant and regularly update their libraries and frameworks to patch any identified vulnerabilities.
The Document Object Model is a fundamental part of both web development and web browsing, especially in the context of JavaScript. Understanding its structure and manipulation methods is essential for creating dynamic and responsive web applications.
However, as highlighted in the Known Vulnerabilities section, developers must also be aware of the potential security risks associated with DOM manipulation. By adopting best practices in security and keeping abreast of vulnerabilities, developers can help ensure their applications remain secure and robust.