· 4 min read
Understanding Parameterized Queries for Secure Database Interaction
Explore the importance of parameterized queries in database management, their security benefits, and best practices across various programming environments.
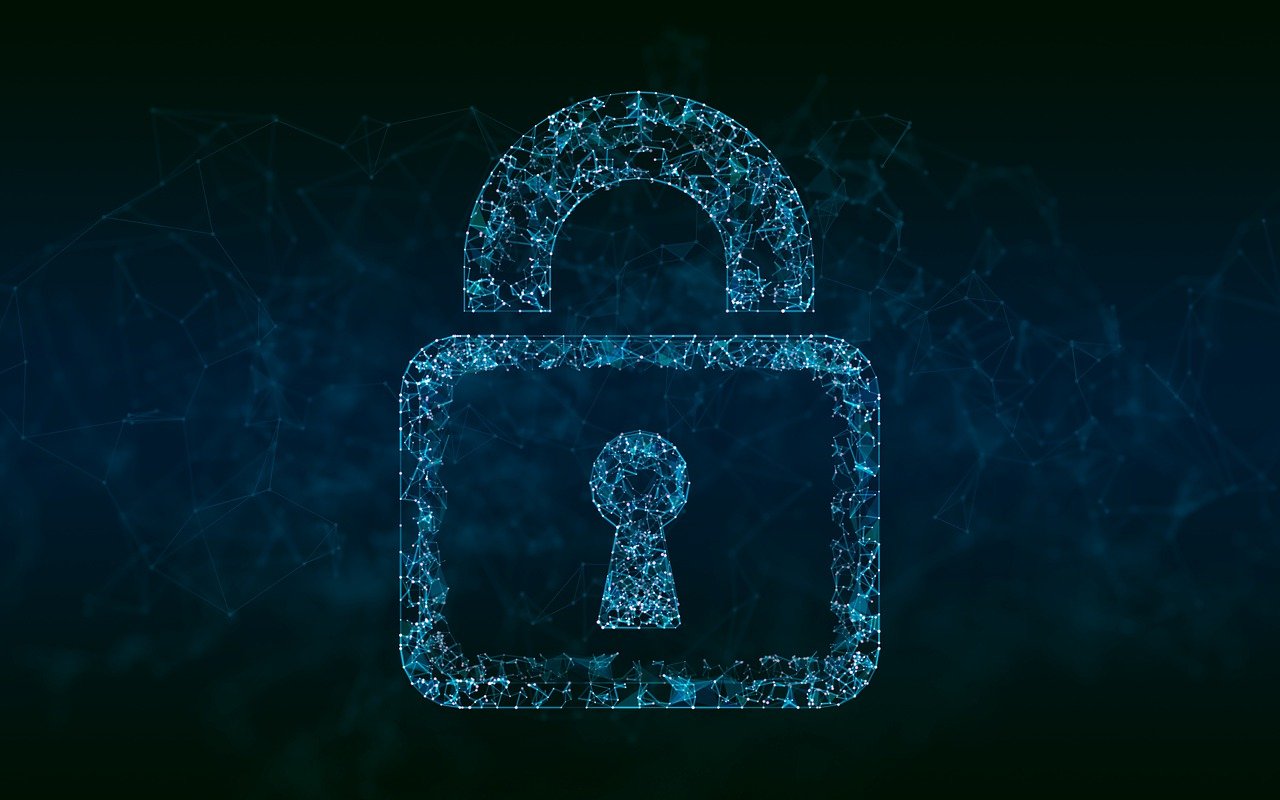
Understanding Parameterized Queries: A Comprehensive Guide
When it comes to database management and application security, the use of parameterized queries plays a vital role. In this article, we’ll delve into what parameterized queries are, how they work, their security implications, and the best practices when using them in various programming environments, such as SQL Server, Java, Python, and others.
What Are Parameterized Queries?
Parameterized queries are a type of SQL query that allow you to execute a statement more securely by separating code from data. This method allows data to be passed to SQL statements in a way that protects against SQL injection attacks. This is a significant improvement over traditional query techniques where user input and SQL statements are combined directly.
What are the Benefits of Using Parameterized Queries?
- Security: By using parameterized queries, you minimize the risk of SQL injections, which are one of the most common vulnerabilities in applications.
- Performance: Many database systems optimize the execution of parameterized queries through caching and reuse. After the query is compiled and executed the first time, subsequent executions can be faster.
- Code Clarity: Parameterized queries can make code easier to read and maintain by clearly defining the structure of the SQL statement and separating logic from variables.
How to Use Parameterized Queries
When you use a parameterized query, you typically define the SQL statement with placeholders for the parameters. You will then provide the actual values separately. For example, in a SQL statement, you might use a placeholder like ?
or @paramName
.
Example in SQL Server
In SQL Server, a simple parameterized query could look like this:
DECLARE @UserID INT;
SET @UserID = 1;
SELECT * FROM Users WHERE ID = @UserID;
This query is not only safe from SQL injection attacks but also clear in its intent.
When to Use a Parameterized Query
Parameterized queries should be used:
- Whenever you execute SQL statements that include user input.
- In scenarios where the structure of the query remains constant, but the data does not.
- Whenever you want to enhance the security of your database interactions.
Parameterized Queries vs. Stored Procedures
While both parameterized queries and stored procedures provide a way to execute SQL statements safely, they are not the same. A stored procedure is a precompiled collection of SQL statements that can take parameters. In contrast, a parameterized query is a single SQL statement that can accept parameters. Stored procedures can incorporate parameterized queries for maximum security and efficiency.
Are Query Parameters Secure?
Security Concerns
One common question is, are query parameters secure? The answer is generally yes, but there are conditions. When used correctly, they help prevent SQL injection, as they treat parameters as data rather than executable code.
However, it�s essential to ensure that:
- You are using a trusted data access library.
- Input validation is also implemented where necessary.
- You handle sensitive data appropriately, as not all database configurations encrypt query parameters by default.
Query Parameters in HTTPS
Are query parameters encrypted in HTTPS? Generally, yes, HTTPS encrypts the entire HTTP request, including the query parameters. However, this does not mean the parameters themselves are secure; rather, HTTPS provides a level of security through encryption during transmission. This does not mitigate the need for careful handling on the server-side.
Implementing Parameterized Queries
SQL Examples
Here’s how you can implement parameterized queries in different databases:
MySQL:
PREPARE stmt FROM 'SELECT * FROM Users WHERE ID = ?'; SET @id = 1; EXECUTE stmt USING @id;
PostgreSQL:
PREPARE my_query (int) AS SELECT * FROM Users WHERE ID = $1; EXECUTE my_query(1);
SQLite:
SELECT * FROM Users WHERE ID = ?;
Programming Languages
Different programming languages have libraries that support parameterized queries. For example:
- In Java, you would use
PreparedStatement
. - In Python, you can use parameterized queries with libraries such as
sqlite3
orpsycopg2
for PostgreSQL.
import sqlite3
connection = sqlite3.connect('database.db')
cursor = connection.cursor()
cursor.execute('SELECT * FROM Users WHERE ID = ?', (user_id,))
In summary, parameterized queries offer a robust mechanism for preventing SQL injection and ensuring that user input is handled safely. Always prefer using parameterized queries when dealing with user data, and consider exploring stored procedures and prepared statements for optimized performance and security.
Understanding and implementing parameterized queries is a crucial skill for developers interacting with databases. By adhering to best practices and leveraging the security features provided by parameterized queries, you can significantly enhance the safety and performance of your applications.